RESTful APIs are the linchpins of modern web and mobile applications, enabling seamless communication between different software systems. This guide aims to be the definitive resource on RESTful APIs, covering everything from basic principles to advanced implementation examples in PHP and Node.js.
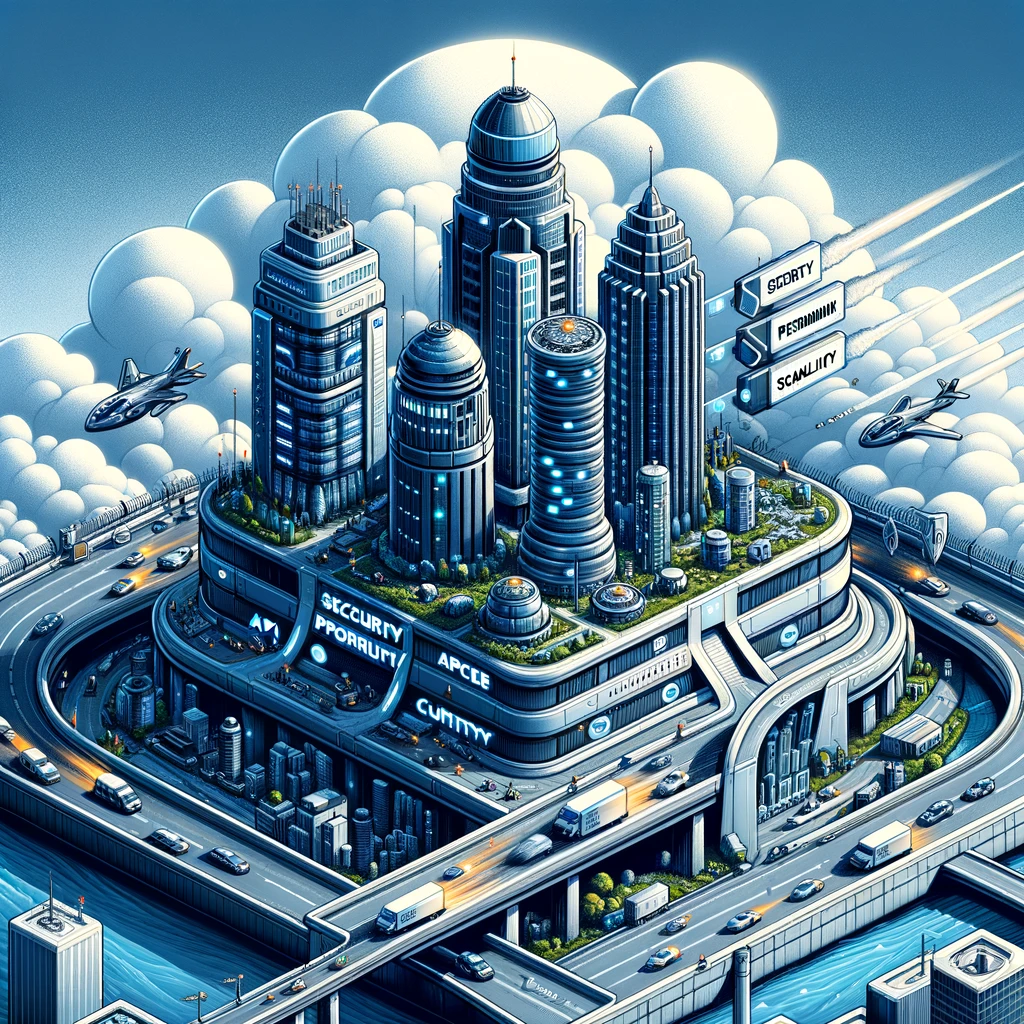
Part 1: Understanding RESTful APIs
What Is a RESTful API?
Imagine you’re using an app to order food from your favorite restaurant. The app communicates with the restaurant’s system to place your order, confirm payment, and update you on the order status. This communication happens over a RESTful API (Representational State Transfer Application Programming Interface), a set of rules that allow programs to communicate over the internet.
RESTful APIs use HTTP requests to perform operations on data, formatted in JSON or XML. These operations are often summarized by the acronym CRUD: Create, Read, Update, and Delete.
Core Principles of REST
- Uniform Interface: A standardized way to interact with the API, ensuring consistency across different parts of the application.
- Stateless: Each request from client to server must contain all the information the server needs to understand and fulfill the request. The server does not store any state about the client session.
- Cacheable: Responses must define themselves as cacheable or not, to prevent clients from reusing stale or inappropriate data.
- Client-Server Architecture: Separating the user interface concerns from the data storage concerns improves the portability of the user interface across multiple platforms and scalability by simplifying server components.
- Layered System: A client cannot ordinarily tell whether it is connected directly to the end server or to an intermediary along the way.
Part 2: Setting Up Your Development Environment
For PHP Development
- Install a Local Server Environment: Download and install XAMPP from https://www.apachefriends.org/index.html. XAMPP will provide you with Apache, MySQL, PHP, and Perl.
- Test Your Installation: Navigate to
http://localhost
in your web browser. If XAMPP is correctly installed, you’ll see the XAMPP dashboard.
For Node.js Development
- Install Node.js: Download and install Node.js from https://nodejs.org/. This will also install npm (Node Package Manager), which is essential for managing Node.js packages.
- Verify Installation: Open your command line or terminal and type
node -v
andnpm -v
to check the installed versions of Node.js and npm, respectively.
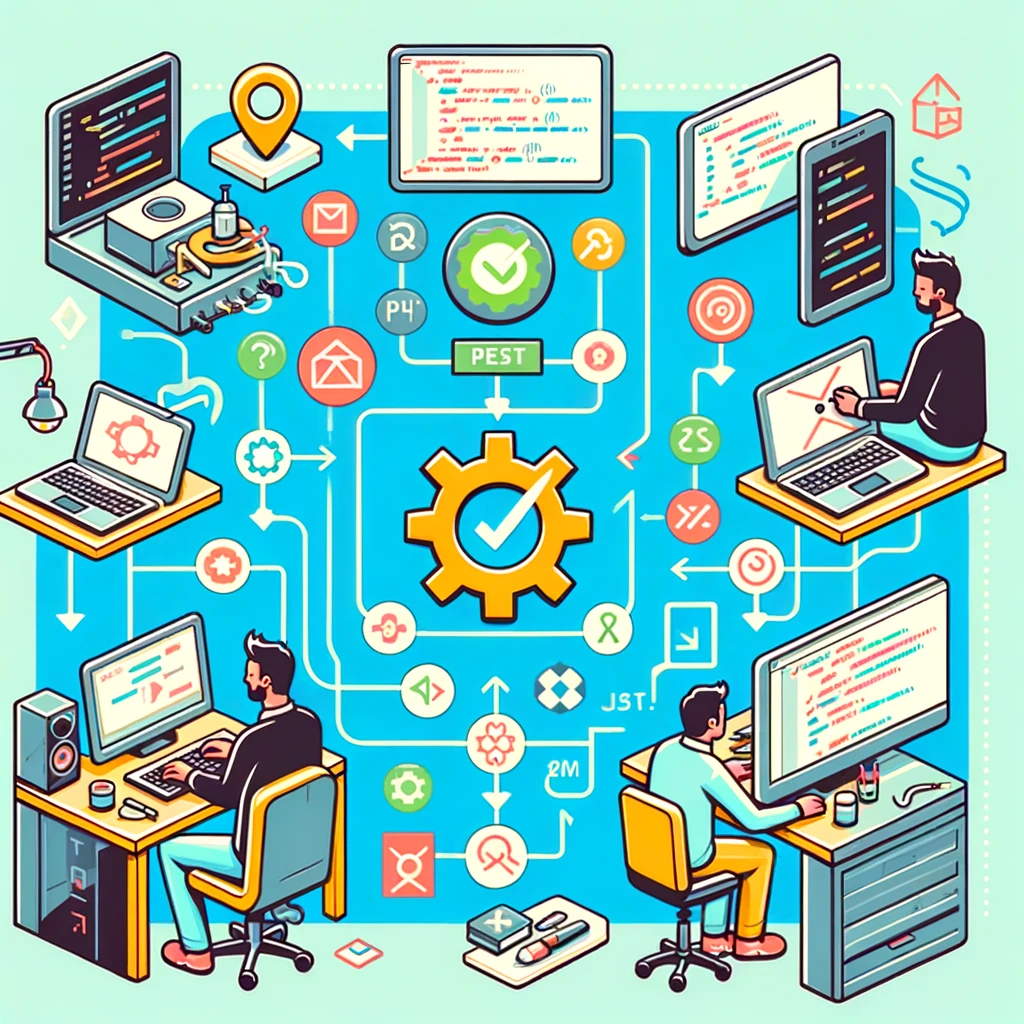
Part 3: Building RESTful APIs with PHP and Node.js
PHP Example 1: A Simple User API
Step 1: Create simpleUserAPI.php
php
<?php
$users = ['Alice', 'Bob', 'Charlie'];
header('Content-Type: application/json');
echo json_encode($users);
?>
Step 2: Test Your API
- Place
simpleUserAPI.php
in thehtdocs
folder of XAMPP. - Access it via
http://localhost/simpleUserAPI.php
.
PHP Example 2: Advanced User Management API
Step 1: Setup MySQL Database
- Create a MySQL database and a
users
table with fieldsid
,name
, andemail
.
Step 2: Create advancedUserAPI.php
php
<?php
include 'database.php'; // Assume you've created a connection in database.php
header("Content-Type: application/json");
$requestMethod = $_SERVER["REQUEST_METHOD"];
switch($requestMethod) {
case 'GET':
$sql = "SELECT * FROM users";
$result = $conn->query($sql);
$users = [];
while($row = $result->fetch_assoc()) {
$users[] = $row;
}
echo json_encode($users);
break;
// Add cases for POST, PUT, DELETE
}
?>
Node.js Example 1: Basic Info API
Step 1: Create infoAPI.js
javascript
const express = require('express');
const app = express();
const PORT = 3000;
app.get('/info', (req, res) => {
res.json({ message: "This is a basic Node.js API" });
});
app.listen(PORT, () => console.log(`Server running on http://localhost:${PORT}`));
Step 2: Run Your API
- Execute
node infoAPI.js
in your terminal.
Node.js Example 2: Detailed User API
Step 1: Install Express and Body-Parser
shell
npm install express body-parser
Step 2: Create detailedUserAPI.js
javascript
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
const PORT = 3000;
let users = [{ id: 1, name: 'Alice', email: 'alice@example.com' }];
app.get('/users', (req, res) => res.json(users));
app.post('/users', (req, res) => {
const newUser = { id: users.length + 1, ...req.body };
users.push(newUser);
res.status(201).send(newUser);
});
app.listen(PORT, () => console.log(`Server running on http://localhost:${PORT}`));
Part 4: Best Practices and Advanced Topics
Security Considerations
- Implement authentication and authorization.
- Use HTTPS to encrypt data in transit.
- Validate and sanitize all input data.
Performance Optimization
- Enable caching where appropriate.
- Limit data payload sizes.
- Use compression to reduce response sizes.
Scalability
- Design your API for scalability from the start.
- Consider using a microservices architecture to allow parts of your system to scale independently.
Documentation and Versioning
- Provide comprehensive API documentation for developers.
- Use versioning to manage changes to your API over time.
Conclusion
Mastering RESTful APIs is a journey that involves understanding foundational principles, practicing with real-world examples, and continuously learning about advanced concepts and best practices. By following the tutorials and advice in this guide, you’re well on your way to becoming proficient in creating and working with RESTful APIs. The path to mastery is through practice and exploration, so don’t hesitate to experiment with the examples provided and explore further into each topic.